nose进行单元测试,和unittest一样,有跳过某个方法,指定特定类进行测试的功能。
识别规则
使用nose进行单元测试,测试用例识别规则如下:
1 2 3 4 5
| nosetests only_test_this.py nosetests test.module nosetests another.test:TestCase.test_method nosetests a.test:TestCase nosetests /path/to/test/file.py:test.function
|
示例
执行全部测试
直接代码示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| #!/usr/bin/env python3 # -*- coding: utf-8 -*- import traceback from nose.tools import eq_ from nose.plugins.attrib import attr from nose.plugins.skip import SkipTest from functools import reduce
class test_nosetests():
#测试加法 def test_sum(self): a = 1 b = 2 res = 3 eq_(a+b,res)
#测试乘法 def test_mul(self): a = 1 b = 2 res = 2 eq_(a*b, res)
#测试除法 def test_div(self): a = 2 b = 0 res = 1 try: eq_(a/b, res) except ZeroDivisionError as e: print(traceback.print_exc())
#测试reduce函数 def test_reduce(self): req = range(1,6) a = 120 res = reduce(lambda x, y: x*y, req) eq_(a, res)
|
想要对test_nosetests
该方法进行测试,可以:
1 2
| 1. nosetests -v NoseTestsexercise.py --with-html --html-file=G:/workstation/report/test_nosetests.html 2. nosetests -v NoseTestsexercise.py:test_nosetests --with-html --html-file=G:/workstation/report/test_nosetests.html
|
由于该python文件只有这一个测试类,所以直接nosetests该python文件即可以进行测试,或者是指定该python文件的测试类如NoseTestsexercise.py:test_nosetests
方式
测试结果: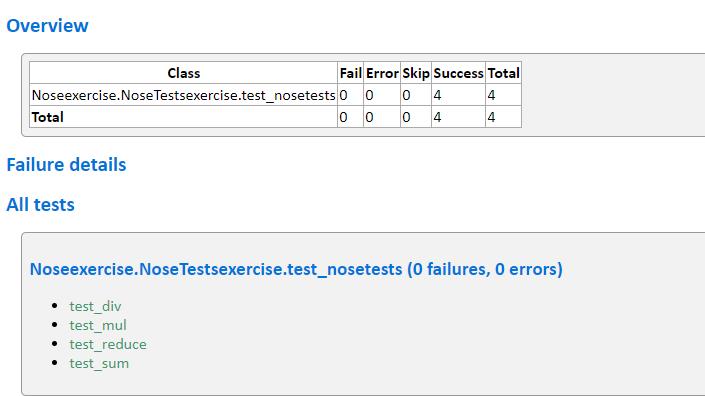
执行指定方法的测试
执行指定的一个方法:
1
| nosetests -v NoseTestsexercise.py:test_nosetests.test_reduce --with-html --html-file=G:/workstation/report/test_nosetests.html
|
示例执行了test_nosetests
类下的test_reduce
方法的测试
测试结果: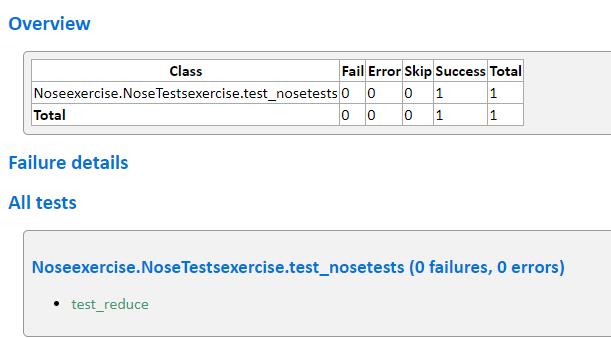
跳过指定方法的测试
有的时候,只需要跳过某个方法:
例如想跳过test_mul
该方法,只需要在代码上增加跳过标志即可
1 2 3 4 5 6 7
| #测试乘法 def test_mul(self): a = 1 b = 2 res = 2 eq_(a*b, res) raise SkipTest
|
执行:
1 2
| nosetests -v NoseTestsexercise.py:test_nosetests --with-html --html-file=G:/workstation/report/test_nosetests.html
|
测试结果: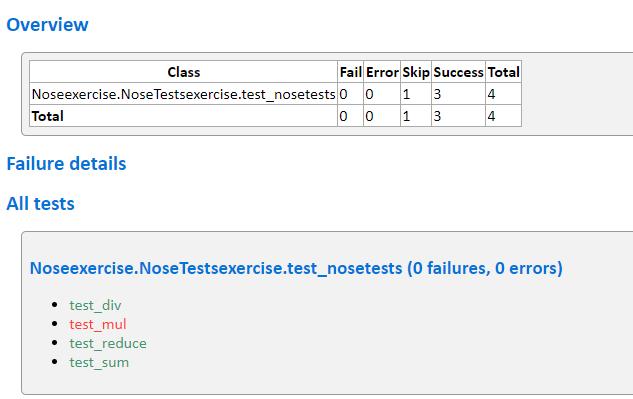
其他
另外,nosetests也有用例执行优先级的功能
1 2 3 4
| from nose.plugins.attrib import attr @attr(speed='slow') def test_big_download(self): print('pass')
|
执行只需要:nosetests -a speed=slow
即可
参考用法